Node.js
- npm
- Node Package Manager
- ejs
- Embedded Java Script
Install express
npm install express
Install ejs
npm install ejs
Check installed packages
npm list express ejs
+-- ejs@3.1.10
`-- express@4.21.2
Rooting
Here's a code to deliver index.ejs file when accessed with http://127.0.0.1:3000/.const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.render('index.ejs');
});
app.get('/home', (req, res) => {
res.render('home.ejs');
});
Template Engine ①
<section>
<h3>Template Engine</h3>
<% const t=["star", "constellation" , "dwarf" , "moon" , "orbit" ] %>
<p>t: <%= t %></p>
<p><%= t.length %></p>
<ul>
<% t.forEach(el=> { %>
<li><%= el %></li>
<% }); %>
</ul>
</section>
Template Engine ②
<head>
<style>
td, th {
border: 1px solid black;
}
th {
text-align: center;
}
td {
text-align: right;
}
td:nth-of-type(1) {
min-width: 40px;
}
td:nth-child(2) {
min-width: 120px;
}
</style>
</head>
<body>
<% const t2 = [ {id: 0, name: "Isac"}, {id: 1, name: "Kate"}, {id: 2, name: "Mable"}, {id: 3, name: "Chris"}, {id: 4, name: "Jun"}] %>
<table style="border: 1px solid black;">
<tr>
<th>id</th>
<th>name</th>
</tr>
<% t2.forEach(el => { %>
<tr>
<td><%= el.id %></td>
<td><%= el.name %></td>
</tr>
<% }); %>
</table>
</body>
The outcome looks like this:
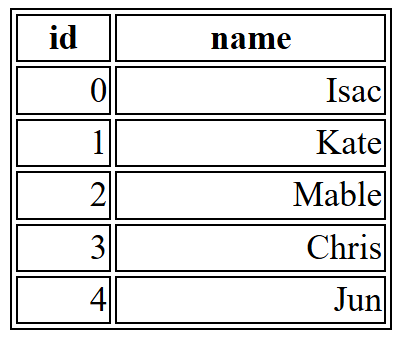